Diving Deep into the Cocktail Sort Algorithm
Also often referred to as the bidirectional bubble sort, cocktail shaker sort, ribbon sort, or even happy hour sort, the cocktail sort algorithm is a remarkable tool for computer programmers. This all-inclusive guide serves to provide a detailed insight into the distinctive characteristics and workings of the cocktail sort algorithm, including its applications, merits, and drawbacks.
Decoding the Logic Behind the Cocktail Sort Algorithm
The cocktail sort algorithm builds upon the bubble sort algorithm. Uniquely, it traverses the list in both directions rather than in just one direction. Its back-and-forth motion is reminiscent of the shaking process associated with making cocktails, hence the suggestive name.
Demystifying the Working Mechanism of the Cocktail Sort Algorithm
The cocktail sort algorithm begins at the opening of the list, scanning each pair of neighboring elements, swapping them if they are in incorrect order. Once the end of the list is met, the algorithm repeats the process, but this time moving in the opposite direction—commencing from the last element and traversing to the first. This cycle continues until no further swaps are necessary, signifying that the list is now ordered.
Breaking Down the Implementation of the Cocktail Sort Algorithm
For a stronger grasp of the cocktail sort algorithm, let’s break down its operations step by step. Starting from the first element, every pair of adjacent items is inspected. If the first element is found to be greater than the second, an immediate swap is performed. This process is repeated until the end of the list is reached. At this point, the algorithm commences the backward pass, comparing the last and second-to-last elements. If the second-to-last item is found to be larger, another swap is performed. This backward process is continued until reaching the start of the list. These steps are repeated until no more swaps occur.
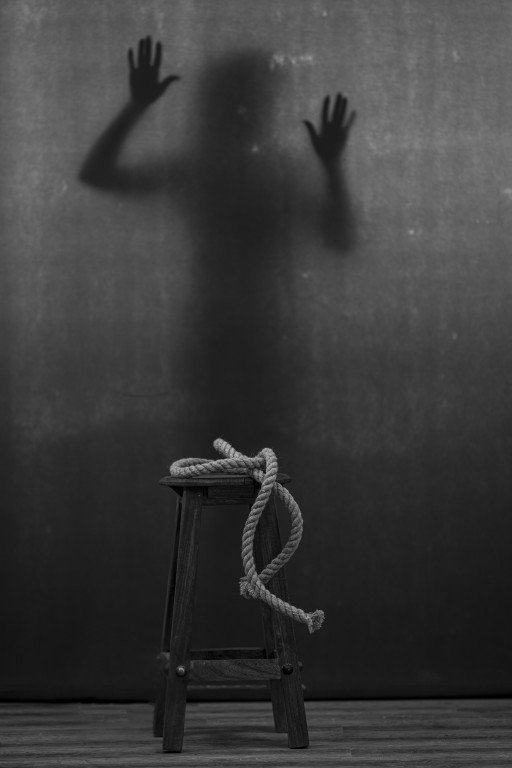
Deconstructing the Time Complexity of the Cocktail Sort Algorithm
Time complexity profoundly influences the analysis of the cocktail sort algorithm. The best-case scenario is when the list is already sorted, it takes linear time—giving an optimal time complexity of O(n). The worst-case scenario entails the smallest or largest element located at either end of the list, which can take a quadratic amount of time to reposition. Hence, we have a time complexity of O(n²) for the worst case. The average-case scenario scenario also yields a quadratic time complexity of O(n²).
Evaluating the Strengths and Weaknesses of the Cocktail Sort Algorithm
Every computational strategy, including the cocktail sort algorithm, comes with its advantages and limitations. It is simple, straightforward, and beneficial for almost sorted lists, making it an excellent resource for programmers at every level. However, the algorithm might seem inefficient for larger lists, and it does not assure stability, which in computational terms, translates to the ability to maintain relative order.
Examining the Practical Applications of the Cocktail Sort Algorithm
Despite its shortcomings, the cocktail sort algorithm finds utility in real-world applications. It proves useful in organising small data lists and near about sorted data lists due to its simple nature and bidirectional mechanism, respectively. The role of the cocktail sort algorithm is further clarified by the comprehensive guide to list data structures.
Visualizing the Cocktail Sort Algorithm Through Python Code
To round off this instructional guide, the following Python code snippet demonstrates the implementation of the cocktail sort algorithm for practical applications.
def cocktailSort(arr):
n = len(arr)
swapped = True
start = 0
end = n-1
while (swapped==True):
swapped = False
for i in range (start, end):
if (arr[i] > arr[i 1]) :
arr[i], arr[i 1]= arr[i 1], arr[i]
swapped=True
if (swapped==False):
break
swapped = False
end = end-1
for i in range(end-1, start-1,-1) :
if (arr[i] > arr[i 1]):
arr[i], arr[i 1] = arr[i 1], arr[i]
swapped = True
start = start 1
In conclusion, the cocktail sort algorithm is simple and handy for sorting small or nearly sorted lists. Developing a thorough understanding of numerous algorithms strengthens the grasp of computational complexity and enhances problem-solving skills.
Enjoy your coding adventures!
Related Posts
- 10 Essential Aspects of Understanding TimSort: The Pinnacle of Sorting Algorithms
- Understanding the Elliptic Curve Cryptography (ECC) Algorithm: A Comprehensive and Detailed Guide
- Understanding Minimum Spanning Trees: 5 Key Insights for Efficiency
- Expansive Guide to Maximizing XGBoost Algorithm: Remarkable Insights and Intricate Details
- 5 Essential Tips for Mastering C Programming Algorithms