Introduction to Python Palindrome Detection
Recognizing palindrome numbers is a captivating concept in programming, where digits mirror each other when reversed. This unique numerical property finds significance in disciplines like computer science and cryptography.
Understanding Palindromes’ Unique Symmetry
Palindromes showcase a mesmerizing bilateral symmetry that echoes with linguistic palindromes such as “deified” or “civic.”
Algorithmic Approach in Python
In Python, verifying palindromic values involves reversing the number and cross-checking it with its original form to validate its palindromic status.
Leveraging Python’s Elegance
The streamlined nature of Python makes it a preferred choice for crafting palindrome verification mechanisms efficiently.
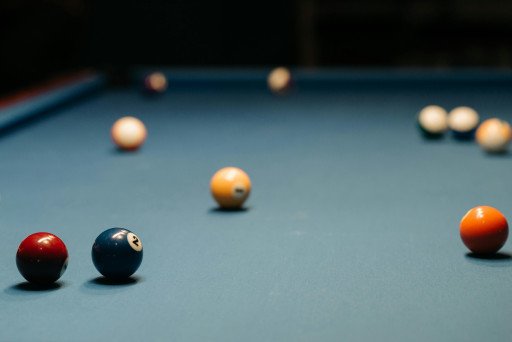
Step-by-Step Palindrome Verification Guide
- Acquire the target number for analysis.
- Transform the number into a string.
- Reverse the string using Python’s slicing syntax.
- Compare the original string with the reversed to affirm its symmetry.
- Report the findings to the user.
Python’s Advanced Solutions for Palindromes
Python affords more intricate solutions due to its rich libraries, enhancing the simplicity and efficiency of the process.
Deconstructing the Basic Palindrome Checker
def is_palindrome(number):
num_str = str(number)
reversed_num_str = num_str[::-1]
return num_str == reversed_num_str
if is_palindrome(12321):
print("The number is a palindrome.")
else:
print("The number is not a palindrome.")
The essential palindrome verification method unfolds in this rudimentary program.
Algorithmic Enhancements
When confronting large datasets or conducting numerous checks, optimizing the code can lead to significant performance gains.
Recursive techniques can be utilized for a more seamless approach, sometimes circumventing explicit conversions.
Optimization via Python Libraries
Python’s itertools
and functools
modules contribute to crafting more concise code without compromising on efficiency.
Recursive Function Example
def is_palindrome_recursive(number, left_index, right_index):
if left_index >= right_index:
return True
if number[left_index] != number[right_index]:
return False
return is_palindrome_recursive(number, left_index + 1, right_index - 1)
def check_palindrome(number):
num_str = str(number)
return is_palindrome_recursive(num_str, 0, len(num_str) - 1)
if check_palindrome(12321):
print("The number is a palindrome.")
else:
print("The number is not a palindrome.")
Custom Python Functions for Special Circumstances
There are instances where palindromes might deviate from typical patterns, necessitating bespoke functions for accurate detection.
Essential Error Handling
Implementing strong error handling ensures the software copes with unexpected input gracefully, enhancing user experience.
Mastering Python Palindrome Detection
Gaining proficiency in palindrome determination with Python paves the way for robust programming capabilities and deeper mathematical insights.
For further mastery in Python, consider exploring the steps outlined in comprehensive python mastery key steps to expertise.
Related Posts
- Python String Manipulation Techniques: A 10-Step Mastery Guide
- Comprehensive Free Python Course: Mastering Python Programming from Scratch
- 10 Ways to Leverage Python in Ethical Hacking: A Complete Guide
- Mastering The Art of Python Color Codes: A Comprehensive Guide
- Comprehensive Python Mastery: 8 Key Steps to Expertise