Introduction to Data Structure Manipulation
In the realm of computer science, queues and stacks represent cornerstone data structures, each operating with unique principles. A queue upholds a ‘first in, first out’ (FIFO) protocol, contrastingly a stack adheres to ‘last in, first out’ (LIFO). Grasping these concepts is paramount when venturing into advanced data handling techniques like Implementing Queue with Two Stacks.
Core Queue Operations Explored
Queues facilitate operations such as ‘enqueue’ to append items and ‘dequeue’ to extract them. These functions are complemented by ‘peek/front’, accessing the foremost element, and ‘isEmpty’, verifying emptiness of the structure.
Stack Operations: The LIFO Paradigm
Stack operations hinge on ‘push’ to place items atop, and ‘pop’ to remove them, supplemented by ‘top/peek’, observing the uppermost item, and ‘isEmpty’, confirming absence of contents.
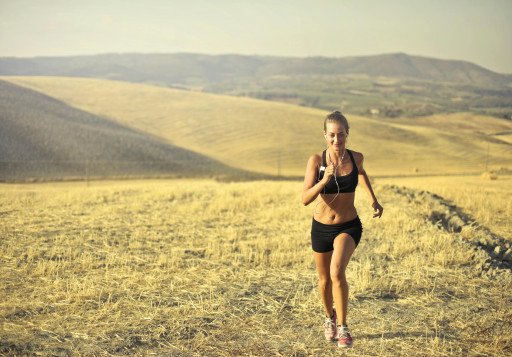
Translating Stacks into Queues
The strategy of Implementing Queue with Two Stacks is intuitive: utilize one stack for incoming entries and another for outgoing. This clever arrangement enables the FIFO sequence through the intrinsic LIFO nature of stacks.
Enqueue Algorithm: Streamlined Ingress
The enqueue procedure is succinct: simply ‘push’ the element onto the first stack, which we shall name ‘stackEnqueue’, thus preserving their original sequence.
Dequeue Algorithm: Strategic Egress
Dequeuing is a multistep affair:
- If ‘stackDequeue’ is populated, ‘pop’ the top.
- Should ‘stackDequeue’ be barren yet ‘stackEnqueue’ is not, invert the pile by transferring elements from ‘stackEnqueue’ to ‘stackDequeue’.
- After the migration, perform the ‘pop’ operation from ‘stackDequeue’.
- An empty dual-stack setup indicates a void queue necessitating error response.
Time Complexity: Amortized Efficiency
The ingenuity of this system delivers an amortized O(1) operation time, as each item is moved merely once within an enqueue-dequeue cycle, despite the O(n) appearance during dequeuing steps.
Utilizing two stacks for a queue’s purpose showcases versatility in parallel computing contexts or where stack-based data access is mandated. Despite increased memory use due to dual structures, the benefits often eclipse drawbacks.
Harnessing Constrained Environments
This technique shines in resource-restricted spaces such as embedded systems, where memory footprint is crucial. It also excels in complex data processing scenarios.
Conclusive Thoughts on Data Structure Flexibility
By Implementing Queue with Two Stacks, we exemplify how to circumvent structural limitations to align with another’s functionality. Such methods fortify foundational knowledge and grant developers the agility to maneuver data effectively in diverse applications.
Embracing and mastering these concepts underscores the critical importance of a programmer’s adaptability. As demands for sophisticated data handling intensify, the ability to creatively leverage data structures becomes indispensable for pioneering solutions.
mastering q learning in python steps intelligent agent
Related Posts
- Understanding the Intricacies of Policy Gradient
- Partitional Clustering: A Comprehensive Guide to Effective Data Segmentation
- An In-depth Guide on Installing XGBoost: Your Key to Machine Learning Success
- Understanding the Number of Connected Components in an Undirected Graph
- 7 Key Advantages of ECC Encryption Efficiency in Modern Cryptography