Delving into Python Data Types
Python, a universally admired programming language, stands out for its simplicity and readability. This makes it a preferred choice for both beginners and experienced developers. The cornerstone of Python’s user-friendliness lies in its basic data types – the fundamental components of coding. Familiarity with these data types is essential for data manipulation and functionality execution in Python.
The Core Python Data Types
In Python, data types categorize data items. They signify the value type, which in turn determines the possible operations on that particular data piece. In this article, we explore Python’s primary data types: strings, integers, floating-point numbers, and booleans.
1. Strings (str)
In Python, a string is a sequence of characters enclosed in single, double, or triple quotes. Strings can encompass letters, numbers, symbols, and whitespace characters.
Construction and Usage of Strings
# Single quotes
greeting = 'Hello, World!'
# Double quotes
person = "John Doe"
# Triple quotes for multi-line strings
multiline_string = '''This is a
multi-line string example.
It spans several lines.'''
String Operations
Strings in Python are immutable, indicating that once formed, the elements within cannot be altered. Yet, they can be concatenated (combined), replicated (duplicated), and sliced (segmented) to create new strings.
# Concatenation
full_greeting = greeting " " person
# Replication
laughter = "Ha" * 3 # Outputs 'HaHaHa'
# Slicing
initial_letter = person[0] # Outputs 'J'
2. Integers (int)
An integer represents a whole number without any fractional component. In Python, integers are used for various arithmetic operations, making them vital for mathematical calculations in programming.
Working with Integers
Basic arithmetic operations with integers in Python are straightforward.
# Addition
total = 7 3 # Outputs 10
# Subtraction
difference = 7 - 3 # Outputs 4
# Multiplication
product = 7 * 3 # Outputs 21
# Division
quotient = 7 / 3 # Outputs 2.333... (returns a float)
3. Floating-Point Numbers (float)
Floating-point numbers, or floats, are numbers with a decimal point. Python uses them to represent real numbers, which includes handling fractions.
Manipulating Floats
Floats behave similarly to integers but permit operations with fractions and decimals.
# Addition with floats
sum_float = 7.5 3.2 # Outputs 10.7
# Rounding floats
rounded_value = round(sum_float, 1) # Outputs 10.7
4. Booleans (bool)
Booleans signify one of two values: True or False. Python uses them mainly to perform conditional checks and control program execution flow.
Boolean Expressions
Boolean values result from comparisons and evaluations within conditions.
# Comparison
is_greater = 7 > 3 # Outputs True
# Logical Operations
is_true = not False # Outputs True
both_true = True and True # Outputs True
either_true = True or False # Outputs True
Advanced Data Types in Python
Beyond the basic data types, Python also features advanced data types like lists, tuples, sets, and dictionaries. These data structures are designed for storing item collections and have their unique set of operations and methods.
1. Lists (list)
Python lists are ordered, mutable item collections. They can accommodate a variety of data types and are defined using square brackets.
Creating and Modifying Lists
# Defining a list
fruits = ['apple', 'banana', 'cherry']
# Adding to a list
fruits.append('orange')
# Removing from a list
fruits.remove('banana')
List Operations
Lists support indexing, slicing, and numerous methods to add, remove, and manipulate items within them.
# Indexing a list
first_fruit = fruits[0] # Outputs 'apple'
# Slicing a list
first_two_fruits = fruits[0:2] # Outputs ['apple', 'cherry']
2. Tuples (tuple)
Tuples are similar to lists, but they are immutable. Once created, a tuple cannot be altered. Tuples are defined using parentheses.
Using Tuples in Python
Tuples are perfect for representing fixed item collections.
# Defining a tuple
tuple_sample = ('python', 3.8, 'version')
# Accessing tuple elements
language = tuple_sample[0] # Outputs 'python'
3. Sets (set)
Sets are unordered collections of unique items. They are mutable and can perform mathematical set operations like union, intersection, and difference.
Creating and Utilizing Sets
# Defining a set
numbers_set = {1, 2, 3, 4, 5}
# Adding an element to a set
numbers_set.add(6)
# Removing an element from a set
numbers_set.discard(3)
4. Dictionaries (dict)
Python dictionaries are collections of key-value pairs. They are mutable and unordered, allowing for quick lookups and direct value access based on their keys.
Dictionary Operations
# Defining a dictionary
person_info = {'name': 'John', 'age': 30, 'city': 'New York'}
# Accessing dictionary values by key
person_name = person_info['name'] # Outputs 'John'
# Adding a new key-value pair
person_info['occupation'] = 'Engineer'
Python Data Type Conversion
Often, data needs to be converted from one type to another – this is called type casting or type conversion. Python provides built-in functions for explicit type conversion.
Data Type Conversions
Conversions between strings, integers, floats, and more can be achieved using Python’s built-in functions.
# Converting integer to string
age = 30
age_str = str(age) # Outputs '30'
# Converting string to float
height_str = "1.85"
height_float = float(height_str) # Outputs 1.85
Wrapping Up: Mastery of Python Data Types for Effective Programming
Comprehending and employing Python’s basic data types is critical to writing effective and efficient programs. By mastering strings, integers, floats, booleans, and the more complex data types like lists, tuples, sets, and dictionaries, programmers can confidently address a wide range of problems. The ability to convert data types further broadens Python coding possibilities, ensuring that developers can craft solutions tailored to any challenge. Check out our unveiled secrets visual basic mastery intensive guide parts for more programming insights.
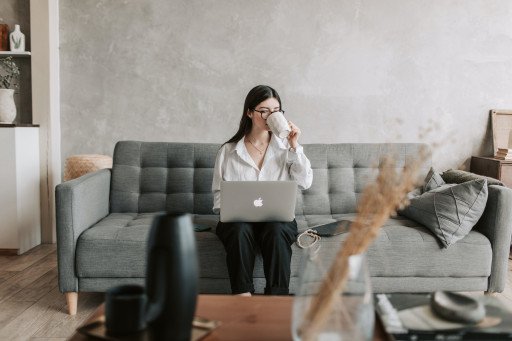
Related Posts
- 10 Key Aspects of Mastering Numberblocks Basics: Boost Your Numeracy Skills Today
- Mastering IF Statements in Visual Basic: 7 Key Insights for Developers
- Mastering Visual Basic Studio: 8 Key Insights for Successful Development
- 6 Techniques for Programming with GW-Basic: A Vintage Coding Guide
- 10 Effective Techniques for Mastering Essential Skills Fast